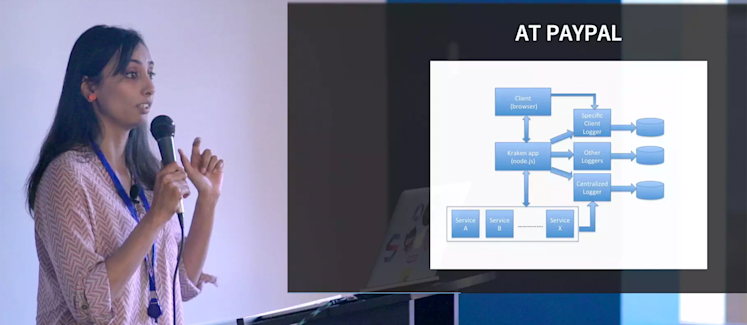
When delivering web applications to a massive user base, resiliency is a forefront consideration. With massive scale applications like PayPal, where millions of users are connected at a given time, scale and orchestration of the app is key. According to Poornima Venkatakrishnan of PayPal, when running at scale, it’s absolutely essential to have:
- Analytics tracking
- Error monitoring and instrumentation
- Experimentation, A/B testing tracking
- Data collection and analysis
In the talk below, Venkatakrishnan covers efforts towards two different aspects of adding resilience to web applications.
The first is logging, which includes a number of requirements including the need to log into various systems, the ability to tag logs, and the ability to propagate data into logger without having to pass ‘req’ around.
The second is circuit breaking to provide stability and prevent cascading failures in distributed systems. Lastly, Venkatakrishnan covers the requirements across Node.js applications, and how we’re looking to solve them.