Transmission Control Protocol vs User Datagram Protocol
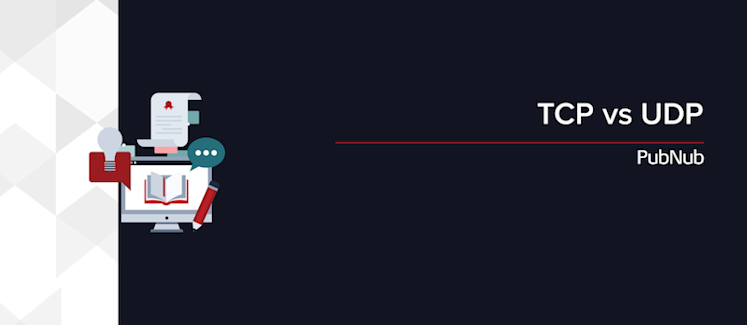
TCP vs UDP Comparison
When developing networked applications, understanding the differences between Transmission Control Protocol (TCP) and User Datagram Protocol (UDP) is crucial. Both are transport layer protocols, but they offer distinct approaches to data transmission. In this guide, we’ll dive into their core differences, advantages, and use cases to help you decide which one fits your application needs.
Overview of TCP and UDP
TCP (Transmission Control Protocol)
TCP is a connection-oriented protocol that ensures reliable data transfer between devices. It breaks data into smaller packets, ensures that these packets are delivered in order, and checks for errors during transmission.
UDP (User Datagram Protocol)
UDP is a connectionless protocol that sends data without establishing a connection. It does not guarantee delivery, order, or error correction, making it faster but less reliable than TCP.
Key Differences Between TCP and UDP
Connection Management
The first major difference between TCP and UDP is that TCP is connection-oriented, while UDP is connectionless. TCP requires a three-step handshake to establish a connection before any data can be transmitted. This process ensures that both the sender and receiver are ready for data exchange. UDP, however, does not establish a connection. It sends data directly without any setup, reducing overhead but also removing any guarantees about delivery.
Reliability
TCP is built to ensure that all data sent by the application is received by the other side, and in the correct order. It uses acknowledgments (ACKs) to confirm receipt of data and retransmits any packets that are lost during transmission. In contrast, UDP does not provide any reliability mechanisms. Once a datagram (packet) is sent, UDP does not check whether it was received or retransmit it if it was lost. As a result, UDP is faster but less reliable than TCP.
Data Transmission
TCP transmits data as a continuous stream, ensuring that the packets are received in the same order they were sent. If packets arrive out of order, TCP will reorder them. UDP, on the other hand, sends data in distinct, independent packets called datagrams. Since there’s no reordering, packets can arrive in any sequence or may even be lost, making UDP suitable for applications that don’t require perfectly ordered data.
Overhead comparison
TCP introduces additional overhead because of its connection management, flow control, and error-checking mechanisms. Each TCP packet includes a larger header (20 to 60 bytes) due to the information required for these features. UDP is more lightweight, with only an 8-byte header, which makes it faster and more efficient for applications that prioritize speed and can tolerate some loss of data.
Flow and Congestion Control
TCP has built-in flow control mechanisms (such as the sliding window technique) to prevent overwhelming the receiver with too much data at once. It also employs congestion control to adjust the transmission rate based on network conditions, reducing traffic when the network is congested. UDP does not have any flow or congestion control mechanisms, which means data is sent at a constant rate regardless of network conditions or the state of the receiver. While this can lead to faster transmission, it also increases the risk of packet loss in congested networks.
When to Use TCP vs UDP
Use TCP When:
Reliability is critical: For applications like file transfers, emails, or web browsing, where accurate data transmission is necessary, TCP ensures that every packet is received correctly and in order.
Data integrity is a priority: Applications that need to ensure that no data is lost or corrupted, such as databases or secure connections, should use TCP.
A consistent connection is needed: Protocols like HTTP, FTP, and SSH require a stable connection to transmit data between clients and servers.
Use UDP When:
Speed is more important than reliability: In real-time applications like online gaming, video streaming, or VoIP, losing some packets is preferable to the delay caused by retransmitting lost packets.
Broadcasting or multicasting: UDP is well-suited for sending data to multiple recipients simultaneously, such as in video conferences or live broadcasts.
Low-latency communication is required: For scenarios where fast data transmission is essential, and slight data loss is acceptable, UDP is ideal.
Real-World Applications of TCP and UDP
TCP Applications:
Web Browsing (HTTP/HTTPS): Ensures the reliable loading of web pages.
File Transfers (FTP): Guarantees that all data is transmitted accurately.
Email Protocols (SMTP, IMAP, POP3): Ensures the complete and correct delivery of email messages.
UDP Applications:
DNS Queries: Allows quick domain name resolution without the need for reliability.
VoIP (Voice over IP): Ensures real-time voice communication, even with occasional packet loss.
Online Gaming: Provides low-latency communication between players, where speed is more important than perfect accuracy.
Implementing TCP and UDP in Code
TCP Client Example (Python)
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('localhost', 8080))
sock.sendall(b'Hello, TCP Server!')
response = sock.recv(1024)
print(f"Received: {response.decode()}")
sock.close()
UDP Client Example (Python)
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.sendto(b'Hello, UDP Server!', ('localhost', 8080))
response, _ = sock.recvfrom(1024)
print(f"Received: {response.decode()}")
sock.close()
Conclusion
Choosing between TCP and UDP depends on the specific needs of your app. If reliability and data integrity are essential, TCP is the best choice. However, if speed and low latency are critical and you can tolerate some data loss, UDP is the way to go. Understanding these differences will help you make the right decision for your application’s performance and reliability requirements.
Happy coding!